SimulIDE 1.1.0 Program Counter crash, 8051
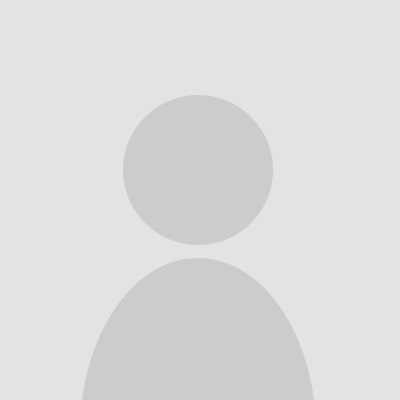
Quote from ivo on October 13, 2024, 3:10 amI get this crash trying to run my 8051 code, using SDCC. It used to work in 1.0.
Overall I want to count using event timer on P3_5, and display count on LCD. Previously, I could never get the event timer to work. Now in 1.1.0 I commented the functional code to only print LCD, but it still crashes, I don't know why?
[spoiler title=""]
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
eMcu::stepCpu: Error PC = 4096 PGM size = 4096
MCU stopped
Simulation Stopped
[/spoiler]
[spoiler title="main.c"]
#include <stc89.h> #include <stdio.h> #include <limits.h> #define Nop() __asm nop __endasm void delay_ms(unsigned int ); void lcd_init(void); void lcd_cmd(unsigned char ); void lcd_char(unsigned char ); void lcd_clear(void); void lcd_home(void); void lcd_string(char* ); void lcd_long(unsigned long); void count_t1_freq(void); int putchar(int c); #define CLEAR 0x01 #define HOME 0x02 #define RS P2_4 #define RW P2_3 #define EN P2_2 #define LCD_DATA P0 #define LED P1_0 #define UF_BTN P3_6 unsigned short duty_on = 0; unsigned short duty_off = 0; unsigned int timer1_of = 0; unsigned long count_result = 0; volatile char counting = 0; void interrupt0_isr(void) __interrupt(IE0_VECTOR) { } void timer0_isr(void) __interrupt(TF0_VECTOR) { // Stop timers TR0 = 0; TR1 = 0; // Calculate result of timer 1 count_result = ((unsigned long)TL1) + (((unsigned long)TH1) << 8); count_result += ((unsigned long)timer1_of) * ((unsigned long)65536); counting = 0; } // Counts overflows void timer1_isr(void) __interrupt(TF1_VECTOR) { timer1_of++; } void count_t1_freq(void) { counting = 1; count_result = 0; // Disable timers TR0 = 0; TR1 = 0; // Enable timer edge interrupts IT0 = 1; IT1 = 1; // Enable overflow interrupts ET0 = 1; ET1 = 1; // Clear gate so TRx controls timers TMOD &= ~T0_GATE; TMOD &= ~T0_GATE; // Set timer modes to 16-bit cascaded TMOD |= T0_M0; TMOD &= ~T0_M1; TMOD |= T1_M0; TMOD &= ~T1_M1; // T0 in timing mode, T1 in counting mode TMOD &= ~T0_CT; TMOD |= T1_CT; // Reset timer counts; TL0 = 0; TH0 = 0; TL1 = 0; TH1 = 0; // Reset overflow timer1_of = 0; // Start timers TR0 = 1; TR1 = 1; } int main(void) { EA = 1; // enable interrupts EX0 = 0; EX1 = 0; // Disable external interrupts IP = 0x02; // timer0 interrupt prio high IPH = 0x02; // timer0 interrupt prio highest lcd_init(); while (1) { lcd_clear(); lcd_string("Counting.."); delay_ms(500); /*count_t1_freq(); while (counting) {} lcd_clear(); lcd_string("c: "); delay_ms(100); lcd_long(count_result); //printf_fast("%d Hz", 5); delay_ms(1000);*/ } } void delay_ms(unsigned int count) { unsigned int i, j; count--; for (i = 0; i < count; i++) { for (j = 0; j < 142; j++) { Nop(); } Nop();Nop();Nop();Nop();Nop(); } } volatile void lcd_init(void) { // Set 8-bit, 8 height font 3 times to ensure correct setting lcd_cmd(0x38); lcd_cmd(0x38); lcd_cmd(0x38); lcd_cmd(0x0C); // Turn on display lcd_clear(); lcd_home(); } void lcd_clear(void) { RS = 0; RW = 0; LCD_DATA = CLEAR; EN = 1; delay_ms(1); EN = 0; delay_ms(4); } void lcd_home(void) { RS = 0; RW = 0; LCD_DATA = HOME; EN = 1; delay_ms(1); EN = 0; delay_ms(4); } void lcd_cmd(unsigned char cmd) { RS = 0; RW = 0; LCD_DATA = cmd; EN = 1; delay_ms(1); EN = 0; delay_ms(2); } int putchar(int c) { lcd_char((unsigned char)c); return c; } void lcd_char(unsigned char data) { RS = 1; RW = 0; LCD_DATA = data; EN = 1; delay_ms(1); EN = 0; delay_ms(2); } void lcd_string(char* str) { while (*str != '\0') { lcd_char(*str); str++; } } void lcd_long(unsigned long num) { unsigned long temp = num / 10; int tens = 1; while (temp > 0) { tens *= 10; temp /= 10; } while (tens > 0) { switch ((num / tens) % 10) { case 0: lcd_char('0'); break; case 1: lcd_char('1'); break; case 2: lcd_char('2'); break; case 3: lcd_char('3'); break; case 4: lcd_char('4'); break; case 5: lcd_char('5'); break; case 6: lcd_char('6'); break; case 7: lcd_char('7'); break; case 8: lcd_char('8'); break; case 9: lcd_char('9'); break; } tens /= 10; } }
[/spoiler]
I get this crash trying to run my 8051 code, using SDCC. It used to work in 1.0.
Overall I want to count using event timer on P3_5, and display count on LCD. Previously, I could never get the event timer to work. Now in 1.1.0 I commented the functional code to only print LCD, but it still crashes, I don't know why?
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
Warning: Simulator::addEvent Repeated event 8051-47-I51Core
eMcu::stepCpu: Error PC = 4096 PGM size = 4096
MCU stopped
Simulation Stopped
#include <stc89.h>
#include <stdio.h>
#include <limits.h>
#define Nop() __asm nop __endasm
void delay_ms(unsigned int );
void lcd_init(void);
void lcd_cmd(unsigned char );
void lcd_char(unsigned char );
void lcd_clear(void);
void lcd_home(void);
void lcd_string(char* );
void lcd_long(unsigned long);
void count_t1_freq(void);
int putchar(int c);
#define CLEAR 0x01
#define HOME 0x02
#define RS P2_4
#define RW P2_3
#define EN P2_2
#define LCD_DATA P0
#define LED P1_0
#define UF_BTN P3_6
unsigned short duty_on = 0;
unsigned short duty_off = 0;
unsigned int timer1_of = 0;
unsigned long count_result = 0;
volatile char counting = 0;
void interrupt0_isr(void) __interrupt(IE0_VECTOR) {
}
void timer0_isr(void) __interrupt(TF0_VECTOR) {
// Stop timers
TR0 = 0; TR1 = 0;
// Calculate result of timer 1
count_result = ((unsigned long)TL1) + (((unsigned long)TH1) << 8);
count_result += ((unsigned long)timer1_of) * ((unsigned long)65536);
counting = 0;
}
// Counts overflows
void timer1_isr(void) __interrupt(TF1_VECTOR) {
timer1_of++;
}
void count_t1_freq(void) {
counting = 1;
count_result = 0;
// Disable timers
TR0 = 0; TR1 = 0;
// Enable timer edge interrupts
IT0 = 1; IT1 = 1;
// Enable overflow interrupts
ET0 = 1; ET1 = 1;
// Clear gate so TRx controls timers
TMOD &= ~T0_GATE;
TMOD &= ~T0_GATE;
// Set timer modes to 16-bit cascaded
TMOD |= T0_M0;
TMOD &= ~T0_M1;
TMOD |= T1_M0;
TMOD &= ~T1_M1;
// T0 in timing mode, T1 in counting mode
TMOD &= ~T0_CT;
TMOD |= T1_CT;
// Reset timer counts;
TL0 = 0; TH0 = 0;
TL1 = 0; TH1 = 0;
// Reset overflow
timer1_of = 0;
// Start timers
TR0 = 1; TR1 = 1;
}
int main(void) {
EA = 1; // enable interrupts
EX0 = 0; EX1 = 0; // Disable external interrupts
IP = 0x02; // timer0 interrupt prio high
IPH = 0x02; // timer0 interrupt prio highest
lcd_init();
while (1) {
lcd_clear();
lcd_string("Counting..");
delay_ms(500);
/*count_t1_freq();
while (counting) {}
lcd_clear();
lcd_string("c: ");
delay_ms(100);
lcd_long(count_result);
//printf_fast("%d Hz", 5);
delay_ms(1000);*/
}
}
void delay_ms(unsigned int count) {
unsigned int i, j;
count--;
for (i = 0; i < count; i++) {
for (j = 0; j < 142; j++) {
Nop();
}
Nop();Nop();Nop();Nop();Nop();
}
}
volatile void lcd_init(void) {
// Set 8-bit, 8 height font 3 times to ensure correct setting
lcd_cmd(0x38);
lcd_cmd(0x38);
lcd_cmd(0x38);
lcd_cmd(0x0C); // Turn on display
lcd_clear();
lcd_home();
}
void lcd_clear(void) {
RS = 0;
RW = 0;
LCD_DATA = CLEAR;
EN = 1; delay_ms(1); EN = 0;
delay_ms(4);
}
void lcd_home(void) {
RS = 0;
RW = 0;
LCD_DATA = HOME;
EN = 1; delay_ms(1); EN = 0;
delay_ms(4);
}
void lcd_cmd(unsigned char cmd) {
RS = 0;
RW = 0;
LCD_DATA = cmd;
EN = 1; delay_ms(1); EN = 0;
delay_ms(2);
}
int putchar(int c) {
lcd_char((unsigned char)c);
return c;
}
void lcd_char(unsigned char data) {
RS = 1;
RW = 0;
LCD_DATA = data;
EN = 1; delay_ms(1); EN = 0;
delay_ms(2);
}
void lcd_string(char* str) {
while (*str != '\0') {
lcd_char(*str);
str++;
}
}
void lcd_long(unsigned long num) {
unsigned long temp = num / 10;
int tens = 1;
while (temp > 0) {
tens *= 10;
temp /= 10;
}
while (tens > 0) {
switch ((num / tens) % 10) {
case 0:
lcd_char('0');
break;
case 1:
lcd_char('1');
break;
case 2:
lcd_char('2');
break;
case 3:
lcd_char('3');
break;
case 4:
lcd_char('4');
break;
case 5:
lcd_char('5');
break;
case 6:
lcd_char('6');
break;
case 7:
lcd_char('7');
break;
case 8:
lcd_char('8');
break;
case 9:
lcd_char('9');
break;
}
tens /= 10;
}
}
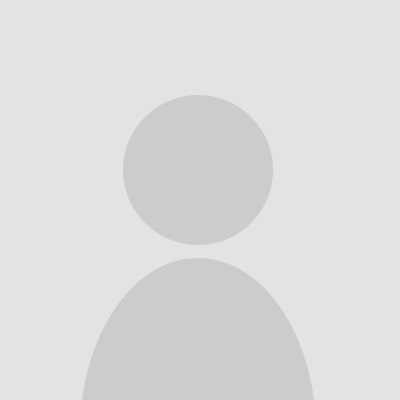
Quote from ivo on October 13, 2024, 3:12 amIf I try to upload my sim1 file, it simply does not appear after clicking submit. I'll paste it text.
[spoiler title="8051.sim1"]
<circuit version="1.1.0-SR0" rev="1917" stepSize="1000000" stepsPS="1000000" NLsteps="100000" reaStep="1000000" animate="0" > <item itemtype="Resistor" CircId="Resistor-34" mainComp="false" ShowProp="Resistance" Show_id="false" Show_Val="true" Pos="-1444,-1008" rotation="0" hflip="1" vflip="1" label="Resistor-34" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="100 Ω" /> <item itemtype="Led" CircId="Led-35" mainComp="false" Show_id="false" Show_Val="false" Pos="-1396,-1008" rotation="0" hflip="-1" vflip="1" label="Led-35" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Color="Red" Grounded="false" Threshold="1.8 V" MaxCurrent="0.03 A" Resistance="0.6 Ω" /> <item itemtype="Ground" CircId="Ground-42" mainComp="false" Show_id="false" Show_Val="false" Pos="-1488,-964" rotation="0" hflip="1" vflip="1" label="Ground-42" idLabPos="-16,8" labelrot="0" valLabPos="-16,20" valLabRot="0" /> <item itemtype="MCU" CircId="8051-47" mainComp="false" Show_id="true" Show_Val="false" Pos="-1312,-1016" rotation="0" hflip="1" vflip="1" label="8051-47" idLabPos="0,-20" labelrot="0" valLabPos="-16,20" valLabRot="0" Frequency="12 MHz" Program="stc89/build/main.hex" Auto_Load="true" varList="TL0,TH0,TL1,TH1,TCON,TMOD" SerialMon="-1" /> <item itemtype="Buffer" CircId="Buffer-54" mainComp="false" Show_id="false" Show_Val="false" Pos="-1348,-1008" rotation="-180" hflip="1" vflip="1" label="Buffer-54" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" /> <item itemtype="Hd44780" CircId="Hd44780-57" mainComp="false" Show_id="true" Show_Val="false" Pos="-1180,-1040" rotation="0" hflip="1" vflip="1" label="Hd44780-57" idLabPos="70,-82" labelrot="0" valLabPos="-16,20" valLabRot="0" Rows="2" Cols="16" /> <item itemtype="Tunnel" CircId="Tunnel-67" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-992" rotation="0" hflip="-1" vflip="1" label="Tunnel-67" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D3" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-68" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-968" rotation="0" hflip="-1" vflip="1" label="Tunnel-68" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D0" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-69" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-1024" rotation="0" hflip="-1" vflip="1" label="Tunnel-69" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D7" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-70" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-976" rotation="0" hflip="-1" vflip="1" label="Tunnel-70" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D1" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-71" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-984" rotation="0" hflip="-1" vflip="1" label="Tunnel-71" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D2" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-72" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-1000" rotation="0" hflip="-1" vflip="1" label="Tunnel-72" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D4" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-73" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-1016" rotation="0" hflip="-1" vflip="1" label="Tunnel-73" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D6" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-74" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-1008" rotation="0" hflip="-1" vflip="1" label="Tunnel-74" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D5" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-83" mainComp="false" Show_id="false" Show_Val="false" Pos="-1116,-992" rotation="-90" hflip="1" vflip="1" label="Tunnel-83" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="EN" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-85" mainComp="false" Show_id="false" Show_Val="false" Pos="-1164,-1024" rotation="-90" hflip="1" vflip="1" label="Tunnel-85" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="RS" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-86" mainComp="false" Show_id="false" Show_Val="false" Pos="-1156,-1024" rotation="-90" hflip="1" vflip="1" label="Tunnel-86" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="RW" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-210" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-952" rotation="0" hflip="-1" vflip="1" label="Tunnel-210" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D6" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-211" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-944" rotation="0" hflip="-1" vflip="1" label="Tunnel-211" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D7" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-212" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-976" rotation="0" hflip="-1" vflip="1" label="Tunnel-212" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D3" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-213" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-984" rotation="0" hflip="-1" vflip="1" label="Tunnel-213" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D2" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-214" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-1000" rotation="0" hflip="-1" vflip="1" label="Tunnel-214" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D0" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-215" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-992" rotation="0" hflip="-1" vflip="1" label="Tunnel-215" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D1" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-216" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-968" rotation="0" hflip="-1" vflip="1" label="Tunnel-216" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D4" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-217" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-960" rotation="0" hflip="-1" vflip="1" label="Tunnel-217" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D5" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-245" mainComp="false" Show_id="false" Show_Val="false" Pos="-1244,-872" rotation="0" hflip="-1" vflip="1" label="Tunnel-245" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="EN" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-246" mainComp="false" Show_id="false" Show_Val="false" Pos="-1244,-880" rotation="0" hflip="-1" vflip="1" label="Tunnel-246" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="RW" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-247" mainComp="false" Show_id="false" Show_Val="false" Pos="-1244,-888" rotation="0" hflip="-1" vflip="1" label="Tunnel-247" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="RS" IsBus="false" /> <item itemtype="Buffer" CircId="Buffer-351" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-1024" rotation="-180" hflip="1" vflip="1" label="Buffer-351" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" /> <item itemtype="Buffer" CircId="Buffer-352" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-1016" rotation="-180" hflip="1" vflip="1" label="Buffer-352" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" /> <item itemtype="Buffer" CircId="Buffer-353" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-1008" rotation="-180" hflip="1" vflip="1" label="Buffer-353" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" /> <item itemtype="Buffer" CircId="Buffer-354" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-1000" rotation="-180" hflip="1" vflip="1" label="Buffer-354" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" /> <item itemtype="Buffer" CircId="Buffer-355" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-992" rotation="-180" hflip="1" vflip="1" label="Buffer-355" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" /> <item itemtype="Buffer" CircId="Buffer-356" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-984" rotation="-180" hflip="1" vflip="1" label="Buffer-356" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" /> <item itemtype="Buffer" CircId="Buffer-357" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-976" rotation="-180" hflip="1" vflip="1" label="Buffer-357" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" /> <item itemtype="Buffer" CircId="Buffer-358" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-968" rotation="-180" hflip="1" vflip="1" label="Buffer-358" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" /> <item itemtype="Tunnel" CircId="Tunnel-431" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-876" rotation="0" hflip="1" vflip="1" label="Tunnel-431" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D5" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-432" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-892" rotation="0" hflip="1" vflip="1" label="Tunnel-432" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D3" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-433" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-900" rotation="0" hflip="1" vflip="1" label="Tunnel-433" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D2" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-434" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-908" rotation="0" hflip="1" vflip="1" label="Tunnel-434" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D1" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-435" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-868" rotation="0" hflip="1" vflip="1" label="Tunnel-435" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D6" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-436" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-884" rotation="0" hflip="1" vflip="1" label="Tunnel-436" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D4" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-437" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-916" rotation="0" hflip="1" vflip="1" label="Tunnel-437" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D0" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-438" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-860" rotation="0" hflip="1" vflip="1" label="Tunnel-438" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D7" IsBus="false" /> <item itemtype="Resistor" CircId="Resistor-484" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-916" rotation="0" hflip="1" vflip="1" label="Resistor-484" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" /> <item itemtype="Resistor" CircId="Resistor-486" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-908" rotation="0" hflip="1" vflip="1" label="Resistor-486" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" /> <item itemtype="Resistor" CircId="Resistor-487" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-900" rotation="0" hflip="1" vflip="1" label="Resistor-487" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" /> <item itemtype="Resistor" CircId="Resistor-488" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-892" rotation="0" hflip="1" vflip="1" label="Resistor-488" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" /> <item itemtype="Resistor" CircId="Resistor-489" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-868" rotation="0" hflip="1" vflip="1" label="Resistor-489" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" /> <item itemtype="Resistor" CircId="Resistor-490" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-860" rotation="0" hflip="1" vflip="1" label="Resistor-490" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" /> <item itemtype="Resistor" CircId="Resistor-491" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-884" rotation="0" hflip="1" vflip="1" label="Resistor-491" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" /> <item itemtype="Resistor" CircId="Resistor-492" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-876" rotation="0" hflip="1" vflip="1" label="Resistor-492" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" /> <item itemtype="Tunnel" CircId="Tunnel-500" mainComp="false" Show_id="false" Show_Val="false" Pos="-1348,-1052" rotation="0" hflip="-1" vflip="1" label="Tunnel-500" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="Vcc" IsBus="false" /> <item itemtype="Rail" CircId="Rail-504" mainComp="false" ShowProp="Voltage" Show_id="false" Show_Val="true" Pos="-1372,-1052" rotation="0" hflip="1" vflip="1" label="Rail-504" idLabPos="-16,-24" labelrot="0" valLabPos="-16,8" valLabRot="0" Voltage="5 V" /> <item itemtype="Ground" CircId="Ground-511" mainComp="false" Show_id="false" Show_Val="false" Pos="-1416,-920" rotation="0" hflip="1" vflip="1" label="Ground-511" idLabPos="-16,8" labelrot="0" valLabPos="-16,20" valLabRot="0" /> <item itemtype="Tunnel" CircId="Tunnel-513" mainComp="false" Show_id="false" Show_Val="false" Pos="-1052,-916" rotation="0" hflip="-1" vflip="1" label="Tunnel-513" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="Vcc" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-595" mainComp="false" Show_id="false" Show_Val="false" Pos="-1328,-928" rotation="0" hflip="1" vflip="1" label="Tunnel-595" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="TX" IsBus="false" /> <item itemtype="Tunnel" CircId="Tunnel-596" mainComp="false" Show_id="false" Show_Val="false" Pos="-1328,-936" rotation="0" hflip="1" vflip="1" label="Tunnel-596" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="RX" IsBus="false" /> <item itemtype="Clock" CircId="Clock-611" mainComp="false" Show_id="false" Show_Val="false" Pos="-1360,-896" rotation="0" hflip="1" vflip="1" label="Clock-611" idLabPos="-64,-24" labelrot="0" valLabPos="-16,8" valLabRot="0" Out="true" Running="true" Voltage="5 V" Freq="22345 Hz" Always_On="true" /> <item itemtype="Oscope" CircId="Oscope-613" mainComp="false" Show_id="false" Show_Val="false" Pos="-1804,-824" rotation="0" hflip="1" vflip="1" label="Oscope-613" idLabPos="-90,-100" labelrot="0" valLabPos="-16,20" valLabRot="0" Basic_X="135 _px" Basic_Y="135 _px" BufferSize="600000 Samples" connectGnd="true" InputImped="10 MΩ" TimDiv="1000000000" TimPos="0,0,0,0," VolDiv="1,1,1,1," Tunnels=",,,," Trigger="4" Filter="0.1 V" Trigger="4" AutoSC="4" Tracks="1" HideCh="false,false,false,false," VolPos="0,0,0,0," /> <item itemtype="FreqMeter" CircId="FreqMeter-614" mainComp="false" Show_id="false" Show_Val="false" Pos="-1280,-800" rotation="0" hflip="1" vflip="1" label="FreqMeter-614" idLabPos="-32,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Filter="0.1 V" /> <item itemtype="Oscope" CircId="Oscope-624" mainComp="false" Show_id="false" Show_Val="false" Pos="-1072,-748" rotation="0" hflip="1" vflip="1" label="Oscope-624" idLabPos="-90,-100" labelrot="0" valLabPos="-16,20" valLabRot="0" Basic_X="135 _px" Basic_Y="135 _px" BufferSize="600000 Samples" connectGnd="true" InputImped="10 MΩ" TimDiv="20000000" TimPos="0,0,0,0," VolDiv="2,2,2,2," Tunnels=",,,," Trigger="0" Filter="1 V" Trigger="0" AutoSC="4" Tracks="1" HideCh="false,false,false,false," VolPos="0,0,0,0," /> <item itemtype="Node" CircId="Node-516" mainComp="false" Pos="-1076,-916" /> <item itemtype="Node" CircId="Node-519" mainComp="false" Pos="-1076,-908" /> <item itemtype="Node" CircId="Node-522" mainComp="false" Pos="-1076,-900" /> <item itemtype="Node" CircId="Node-525" mainComp="false" Pos="-1076,-892" /> <item itemtype="Node" CircId="Node-528" mainComp="false" Pos="-1076,-884" /> <item itemtype="Node" CircId="Node-531" mainComp="false" Pos="-1076,-876" /> <item itemtype="Node" CircId="Node-534" mainComp="false" Pos="-1076,-868" /> <item itemtype="Node" CircId="Node-616" mainComp="false" Pos="-1336,-896" /> <item itemtype="Node" CircId="Node-626" mainComp="false" Pos="-1336,-832" /> <item itemtype="Connector" uid="connector-535" startpinid="Resistor-34-rPin" endpinid="Led-35-rPin" pointList="-1428,-1008,-1412,-1008" /> <item itemtype="Connector" uid="connector-536" startpinid="Ground-42-Gnd" endpinid="Resistor-34-lPin" pointList="-1488,-980,-1488,-1008,-1460,-1008" /> <item itemtype="Connector" uid="connector-537" startpinid="8051-47-PORT10" endpinid="Buffer-54-in0" pointList="-1320,-1008,-1332,-1008" /> <item itemtype="Connector" uid="connector-538" startpinid="Buffer-54-out" endpinid="Led-35-lPin" pointList="-1364,-1008,-1380,-1008" /> <item itemtype="Connector" uid="connector-539" startpinid="Hd44780-57-PinEn" endpinid="Tunnel-83-pin" pointList="-1148,-1032,-1148,-992,-1116,-992" /> <item itemtype="Connector" uid="connector-540" startpinid="Tunnel-86-pin" endpinid="Hd44780-57-PinRW" pointList="-1156,-1024,-1156,-1032" /> <item itemtype="Connector" uid="connector-541" startpinid="Tunnel-85-pin" endpinid="Hd44780-57-PinRS" pointList="-1164,-1024,-1164,-1032" /> <item itemtype="Connector" uid="connector-542" startpinid="Tunnel-214-pin" endpinid="8051-47-PORT00" pointList="-1240,-1000,-1256,-1000" /> <item itemtype="Connector" uid="connector-543" startpinid="8051-47-PORT01" endpinid="Tunnel-215-pin" pointList="-1256,-992,-1240,-992" /> <item itemtype="Connector" uid="connector-544" startpinid="Tunnel-213-pin" endpinid="8051-47-PORT02" pointList="-1240,-984,-1256,-984" /> <item itemtype="Connector" uid="connector-545" startpinid="8051-47-PORT03" endpinid="Tunnel-212-pin" pointList="-1256,-976,-1240,-976" /> <item itemtype="Connector" uid="connector-546" startpinid="Tunnel-216-pin" endpinid="8051-47-PORT04" pointList="-1240,-968,-1256,-968" /> <item itemtype="Connector" uid="connector-547" startpinid="8051-47-PORT05" endpinid="Tunnel-217-pin" pointList="-1256,-960,-1240,-960" /> <item itemtype="Connector" uid="connector-548" startpinid="Tunnel-210-pin" endpinid="8051-47-PORT06" pointList="-1240,-952,-1256,-952" /> <item itemtype="Connector" uid="connector-549" startpinid="8051-47-PORT07" endpinid="Tunnel-211-pin" pointList="-1256,-944,-1240,-944" /> <item itemtype="Connector" uid="connector-550" startpinid="8051-47-PORT24" endpinid="Tunnel-247-pin" pointList="-1256,-888,-1244,-888" /> <item itemtype="Connector" uid="connector-551" startpinid="Tunnel-246-pin" endpinid="8051-47-PORT23" pointList="-1244,-880,-1256,-880" /> <item itemtype="Connector" uid="connector-552" startpinid="8051-47-PORT22" endpinid="Tunnel-245-pin" pointList="-1256,-872,-1244,-872" /> <item itemtype="Connector" uid="connector-553" startpinid="Tunnel-68-pin" endpinid="Buffer-358-in0" pointList="-972,-968,-988,-968" /> <item itemtype="Connector" uid="connector-554" startpinid="Tunnel-72-pin" endpinid="Buffer-354-in0" pointList="-972,-1000,-988,-1000" /> <item itemtype="Connector" uid="connector-555" startpinid="Buffer-355-in0" endpinid="Tunnel-67-pin" pointList="-988,-992,-972,-992" /> <item itemtype="Connector" uid="connector-556" startpinid="Tunnel-71-pin" endpinid="Buffer-356-in0" pointList="-972,-984,-988,-984" /> <item itemtype="Connector" uid="connector-557" startpinid="Buffer-357-in0" endpinid="Tunnel-70-pin" pointList="-988,-976,-972,-976" /> <item itemtype="Connector" uid="connector-558" startpinid="Tunnel-74-pin" endpinid="Buffer-353-in0" pointList="-972,-1008,-988,-1008" /> <item itemtype="Connector" uid="connector-559" startpinid="Buffer-352-in0" endpinid="Tunnel-73-pin" pointList="-988,-1016,-972,-1016" /> <item itemtype="Connector" uid="connector-560" startpinid="Tunnel-69-pin" endpinid="Buffer-351-in0" pointList="-972,-1024,-988,-1024" /> <item itemtype="Connector" uid="connector-561" startpinid="Buffer-351-out" endpinid="Hd44780-57-dataPin7" pointList="-1020,-1024,-1084,-1024,-1084,-1032" /> <item itemtype="Connector" uid="connector-562" startpinid="Buffer-352-out" endpinid="Hd44780-57-dataPin6" pointList="-1020,-1016,-1092,-1016,-1092,-1032" /> <item itemtype="Connector" uid="connector-563" startpinid="Buffer-353-out" endpinid="Hd44780-57-dataPin5" pointList="-1020,-1008,-1100,-1008,-1100,-1032" /> <item itemtype="Connector" uid="connector-564" startpinid="Buffer-354-out" endpinid="Hd44780-57-dataPin4" pointList="-1020,-1000,-1108,-1000,-1108,-1032" /> <item itemtype="Connector" uid="connector-565" startpinid="Buffer-355-out" endpinid="Hd44780-57-dataPin3" pointList="-1020,-992,-1116,-992,-1116,-1032" /> <item itemtype="Connector" uid="connector-566" startpinid="Buffer-356-out" endpinid="Hd44780-57-dataPin2" pointList="-1020,-984,-1124,-984,-1124,-1032" /> <item itemtype="Connector" uid="connector-567" startpinid="Buffer-357-out" endpinid="Hd44780-57-dataPin1" pointList="-1020,-976,-1132,-976,-1132,-1032" /> <item itemtype="Connector" uid="connector-568" startpinid="Buffer-358-out" endpinid="Hd44780-57-dataPin0" pointList="-1020,-968,-1140,-968,-1140,-1032" /> <item itemtype="Connector" uid="connector-569" startpinid="Tunnel-437-pin" endpinid="Resistor-484-lPin" pointList="-1132,-916,-1120,-916" /> <item itemtype="Connector" uid="connector-570" startpinid="Tunnel-434-pin" endpinid="Resistor-486-lPin" pointList="-1132,-908,-1120,-908" /> <item itemtype="Connector" uid="connector-571" startpinid="Tunnel-433-pin" endpinid="Resistor-487-lPin" pointList="-1132,-900,-1120,-900" /> <item itemtype="Connector" uid="connector-572" startpinid="Tunnel-432-pin" endpinid="Resistor-488-lPin" pointList="-1132,-892,-1120,-892" /> <item itemtype="Connector" uid="connector-573" startpinid="Tunnel-436-pin" endpinid="Resistor-491-lPin" pointList="-1132,-884,-1120,-884" /> <item itemtype="Connector" uid="connector-574" startpinid="Tunnel-431-pin" endpinid="Resistor-492-lPin" pointList="-1132,-876,-1120,-876" /> <item itemtype="Connector" uid="connector-575" startpinid="Tunnel-435-pin" endpinid="Resistor-489-lPin" pointList="-1132,-868,-1120,-868" /> <item itemtype="Connector" uid="connector-576" startpinid="Tunnel-438-pin" endpinid="Resistor-490-lPin" pointList="-1132,-860,-1120,-860" /> <item itemtype="Connector" uid="connector-577" startpinid="Rail-504-outnod" endpinid="Tunnel-500-pin" pointList="-1356,-1052,-1348,-1052" /> <item itemtype="Connector" uid="connector-578" startpinid="8051-47-RST" endpinid="Ground-511-Gnd" pointList="-1320,-944,-1416,-944,-1416,-936" /> <item itemtype="Connector" uid="connector-579" startpinid="Resistor-484-rPin" endpinid="Node-516-0" pointList="-1088,-916,-1076,-916" /> <item itemtype="Connector" uid="connector-580" startpinid="Resistor-486-rPin" endpinid="Node-519-0" pointList="-1088,-908,-1076,-908" /> <item itemtype="Connector" uid="connector-581" startpinid="Node-516-2" endpinid="Tunnel-513-pin" pointList="-1076,-916,-1052,-916" /> <item itemtype="Connector" uid="connector-582" startpinid="Resistor-487-rPin" endpinid="Node-522-0" pointList="-1088,-900,-1076,-900" /> <item itemtype="Connector" uid="connector-583" startpinid="Node-519-2" endpinid="Node-516-1" pointList="-1076,-908,-1076,-916" /> <item itemtype="Connector" uid="connector-584" startpinid="Resistor-488-rPin" endpinid="Node-525-0" pointList="-1088,-892,-1076,-892" /> <item itemtype="Connector" uid="connector-585" startpinid="Node-522-2" endpinid="Node-519-1" pointList="-1076,-900,-1076,-908" /> <item itemtype="Connector" uid="connector-586" startpinid="Resistor-491-rPin" endpinid="Node-528-0" pointList="-1088,-884,-1076,-884" /> <item itemtype="Connector" uid="connector-587" startpinid="Node-525-2" endpinid="Node-522-1" pointList="-1076,-892,-1076,-900" /> <item itemtype="Connector" uid="connector-588" startpinid="Resistor-492-rPin" endpinid="Node-531-0" pointList="-1088,-876,-1076,-876" /> <item itemtype="Connector" uid="connector-589" startpinid="Node-528-2" endpinid="Node-525-1" pointList="-1076,-884,-1076,-892" /> <item itemtype="Connector" uid="connector-590" startpinid="Resistor-489-rPin" endpinid="Node-534-0" pointList="-1088,-868,-1076,-868" /> <item itemtype="Connector" uid="connector-591" startpinid="Node-531-2" endpinid="Node-528-1" pointList="-1076,-876,-1076,-884" /> <item itemtype="Connector" uid="connector-592" startpinid="Resistor-490-rPin" endpinid="Node-534-1" pointList="-1088,-860,-1076,-860,-1076,-868" /> <item itemtype="Connector" uid="connector-593" startpinid="Node-534-2" endpinid="Node-531-1" pointList="-1076,-868,-1076,-876" /> <item itemtype="Connector" uid="Connector-597" startpinid="Tunnel-596-pin" endpinid="8051-47-PORT30" pointList="-1328,-936,-1320,-936" /> <item itemtype="Connector" uid="Connector-598" startpinid="8051-47-PORT31" endpinid="Tunnel-595-pin" pointList="-1320,-928,-1328,-928" /> <item itemtype="Connector" uid="Connector-612" startpinid="Clock-611-outnod" endpinid="Node-616-0" pointList="-1344,-896,-1336,-896" /> <item itemtype="Connector" uid="Connector-617" startpinid="Node-616-2" endpinid="8051-47-PORT35" pointList="-1336,-896,-1320,-896" /> <item itemtype="Connector" uid="Connector-625" startpinid="Oscope-624-Pin0" endpinid="Node-626-1" pointList="-1160,-796,-1208,-796,-1208,-768,-1336,-768,-1336,-832" /> <item itemtype="Connector" uid="Connector-627" startpinid="Node-626-2" endpinid="Node-616-1" pointList="-1336,-832,-1336,-896" /> <item itemtype="Connector" uid="Connector-615" startpinid="FreqMeter-614-lPin" endpinid="Node-626-0" pointList="-1320,-800,-1320,-832,-1336,-832" /> </circuit>
[/spoiler]
If I try to upload my sim1 file, it simply does not appear after clicking submit. I'll paste it text.
<circuit version="1.1.0-SR0" rev="1917" stepSize="1000000" stepsPS="1000000" NLsteps="100000" reaStep="1000000" animate="0" >
<item itemtype="Resistor" CircId="Resistor-34" mainComp="false" ShowProp="Resistance" Show_id="false" Show_Val="true" Pos="-1444,-1008" rotation="0" hflip="1" vflip="1" label="Resistor-34" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="100 Ω" />
<item itemtype="Led" CircId="Led-35" mainComp="false" Show_id="false" Show_Val="false" Pos="-1396,-1008" rotation="0" hflip="-1" vflip="1" label="Led-35" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Color="Red" Grounded="false" Threshold="1.8 V" MaxCurrent="0.03 A" Resistance="0.6 Ω" />
<item itemtype="Ground" CircId="Ground-42" mainComp="false" Show_id="false" Show_Val="false" Pos="-1488,-964" rotation="0" hflip="1" vflip="1" label="Ground-42" idLabPos="-16,8" labelrot="0" valLabPos="-16,20" valLabRot="0" />
<item itemtype="MCU" CircId="8051-47" mainComp="false" Show_id="true" Show_Val="false" Pos="-1312,-1016" rotation="0" hflip="1" vflip="1" label="8051-47" idLabPos="0,-20" labelrot="0" valLabPos="-16,20" valLabRot="0" Frequency="12 MHz" Program="stc89/build/main.hex" Auto_Load="true" varList="TL0,TH0,TL1,TH1,TCON,TMOD" SerialMon="-1" />
<item itemtype="Buffer" CircId="Buffer-54" mainComp="false" Show_id="false" Show_Val="false" Pos="-1348,-1008" rotation="-180" hflip="1" vflip="1" label="Buffer-54" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" />
<item itemtype="Hd44780" CircId="Hd44780-57" mainComp="false" Show_id="true" Show_Val="false" Pos="-1180,-1040" rotation="0" hflip="1" vflip="1" label="Hd44780-57" idLabPos="70,-82" labelrot="0" valLabPos="-16,20" valLabRot="0" Rows="2" Cols="16" />
<item itemtype="Tunnel" CircId="Tunnel-67" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-992" rotation="0" hflip="-1" vflip="1" label="Tunnel-67" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D3" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-68" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-968" rotation="0" hflip="-1" vflip="1" label="Tunnel-68" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D0" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-69" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-1024" rotation="0" hflip="-1" vflip="1" label="Tunnel-69" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D7" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-70" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-976" rotation="0" hflip="-1" vflip="1" label="Tunnel-70" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D1" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-71" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-984" rotation="0" hflip="-1" vflip="1" label="Tunnel-71" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D2" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-72" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-1000" rotation="0" hflip="-1" vflip="1" label="Tunnel-72" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D4" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-73" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-1016" rotation="0" hflip="-1" vflip="1" label="Tunnel-73" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D6" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-74" mainComp="false" Show_id="false" Show_Val="false" Pos="-972,-1008" rotation="0" hflip="-1" vflip="1" label="Tunnel-74" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D5" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-83" mainComp="false" Show_id="false" Show_Val="false" Pos="-1116,-992" rotation="-90" hflip="1" vflip="1" label="Tunnel-83" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="EN" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-85" mainComp="false" Show_id="false" Show_Val="false" Pos="-1164,-1024" rotation="-90" hflip="1" vflip="1" label="Tunnel-85" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="RS" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-86" mainComp="false" Show_id="false" Show_Val="false" Pos="-1156,-1024" rotation="-90" hflip="1" vflip="1" label="Tunnel-86" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="RW" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-210" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-952" rotation="0" hflip="-1" vflip="1" label="Tunnel-210" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D6" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-211" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-944" rotation="0" hflip="-1" vflip="1" label="Tunnel-211" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D7" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-212" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-976" rotation="0" hflip="-1" vflip="1" label="Tunnel-212" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D3" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-213" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-984" rotation="0" hflip="-1" vflip="1" label="Tunnel-213" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D2" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-214" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-1000" rotation="0" hflip="-1" vflip="1" label="Tunnel-214" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D0" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-215" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-992" rotation="0" hflip="-1" vflip="1" label="Tunnel-215" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D1" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-216" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-968" rotation="0" hflip="-1" vflip="1" label="Tunnel-216" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D4" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-217" mainComp="false" Show_id="false" Show_Val="false" Pos="-1240,-960" rotation="0" hflip="-1" vflip="1" label="Tunnel-217" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D5" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-245" mainComp="false" Show_id="false" Show_Val="false" Pos="-1244,-872" rotation="0" hflip="-1" vflip="1" label="Tunnel-245" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="EN" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-246" mainComp="false" Show_id="false" Show_Val="false" Pos="-1244,-880" rotation="0" hflip="-1" vflip="1" label="Tunnel-246" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="RW" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-247" mainComp="false" Show_id="false" Show_Val="false" Pos="-1244,-888" rotation="0" hflip="-1" vflip="1" label="Tunnel-247" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="RS" IsBus="false" />
<item itemtype="Buffer" CircId="Buffer-351" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-1024" rotation="-180" hflip="1" vflip="1" label="Buffer-351" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" />
<item itemtype="Buffer" CircId="Buffer-352" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-1016" rotation="-180" hflip="1" vflip="1" label="Buffer-352" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" />
<item itemtype="Buffer" CircId="Buffer-353" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-1008" rotation="-180" hflip="1" vflip="1" label="Buffer-353" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" />
<item itemtype="Buffer" CircId="Buffer-354" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-1000" rotation="-180" hflip="1" vflip="1" label="Buffer-354" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" />
<item itemtype="Buffer" CircId="Buffer-355" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-992" rotation="-180" hflip="1" vflip="1" label="Buffer-355" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" />
<item itemtype="Buffer" CircId="Buffer-356" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-984" rotation="-180" hflip="1" vflip="1" label="Buffer-356" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" />
<item itemtype="Buffer" CircId="Buffer-357" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-976" rotation="-180" hflip="1" vflip="1" label="Buffer-357" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" />
<item itemtype="Buffer" CircId="Buffer-358" mainComp="false" Show_id="false" Show_Val="false" Pos="-1004,-968" rotation="-180" hflip="1" vflip="1" label="Buffer-358" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Input_High_V="2.5 V" Input_Low_V="2.5 V" Input_Imped="1000 MΩ" Invert_Inputs="false" Out_High_V="5 V" Out_Low_V="0 V" Out_Imped="40 Ω" initHigh="false" Inverted="false" Open_Collector="false" Tristate="false" Tpd_ps="10000 ps" Tr_ps="3000 ps" Tf_ps="4000 ps" />
<item itemtype="Tunnel" CircId="Tunnel-431" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-876" rotation="0" hflip="1" vflip="1" label="Tunnel-431" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D5" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-432" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-892" rotation="0" hflip="1" vflip="1" label="Tunnel-432" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D3" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-433" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-900" rotation="0" hflip="1" vflip="1" label="Tunnel-433" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D2" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-434" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-908" rotation="0" hflip="1" vflip="1" label="Tunnel-434" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D1" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-435" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-868" rotation="0" hflip="1" vflip="1" label="Tunnel-435" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D6" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-436" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-884" rotation="0" hflip="1" vflip="1" label="Tunnel-436" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D4" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-437" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-916" rotation="0" hflip="1" vflip="1" label="Tunnel-437" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D0" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-438" mainComp="false" Show_id="false" Show_Val="false" Pos="-1132,-860" rotation="0" hflip="1" vflip="1" label="Tunnel-438" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="D7" IsBus="false" />
<item itemtype="Resistor" CircId="Resistor-484" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-916" rotation="0" hflip="1" vflip="1" label="Resistor-484" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" />
<item itemtype="Resistor" CircId="Resistor-486" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-908" rotation="0" hflip="1" vflip="1" label="Resistor-486" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" />
<item itemtype="Resistor" CircId="Resistor-487" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-900" rotation="0" hflip="1" vflip="1" label="Resistor-487" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" />
<item itemtype="Resistor" CircId="Resistor-488" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-892" rotation="0" hflip="1" vflip="1" label="Resistor-488" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" />
<item itemtype="Resistor" CircId="Resistor-489" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-868" rotation="0" hflip="1" vflip="1" label="Resistor-489" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" />
<item itemtype="Resistor" CircId="Resistor-490" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-860" rotation="0" hflip="1" vflip="1" label="Resistor-490" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" />
<item itemtype="Resistor" CircId="Resistor-491" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-884" rotation="0" hflip="1" vflip="1" label="Resistor-491" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" />
<item itemtype="Resistor" CircId="Resistor-492" mainComp="false" Show_id="false" Show_Val="false" Pos="-1104,-876" rotation="0" hflip="1" vflip="1" label="Resistor-492" idLabPos="-16,-24" labelrot="0" valLabPos="-16,6" valLabRot="0" Resistance="10 kΩ" />
<item itemtype="Tunnel" CircId="Tunnel-500" mainComp="false" Show_id="false" Show_Val="false" Pos="-1348,-1052" rotation="0" hflip="-1" vflip="1" label="Tunnel-500" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="Vcc" IsBus="false" />
<item itemtype="Rail" CircId="Rail-504" mainComp="false" ShowProp="Voltage" Show_id="false" Show_Val="true" Pos="-1372,-1052" rotation="0" hflip="1" vflip="1" label="Rail-504" idLabPos="-16,-24" labelrot="0" valLabPos="-16,8" valLabRot="0" Voltage="5 V" />
<item itemtype="Ground" CircId="Ground-511" mainComp="false" Show_id="false" Show_Val="false" Pos="-1416,-920" rotation="0" hflip="1" vflip="1" label="Ground-511" idLabPos="-16,8" labelrot="0" valLabPos="-16,20" valLabRot="0" />
<item itemtype="Tunnel" CircId="Tunnel-513" mainComp="false" Show_id="false" Show_Val="false" Pos="-1052,-916" rotation="0" hflip="-1" vflip="1" label="Tunnel-513" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="Vcc" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-595" mainComp="false" Show_id="false" Show_Val="false" Pos="-1328,-928" rotation="0" hflip="1" vflip="1" label="Tunnel-595" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="TX" IsBus="false" />
<item itemtype="Tunnel" CircId="Tunnel-596" mainComp="false" Show_id="false" Show_Val="false" Pos="-1328,-936" rotation="0" hflip="1" vflip="1" label="Tunnel-596" idLabPos="-16,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Name="RX" IsBus="false" />
<item itemtype="Clock" CircId="Clock-611" mainComp="false" Show_id="false" Show_Val="false" Pos="-1360,-896" rotation="0" hflip="1" vflip="1" label="Clock-611" idLabPos="-64,-24" labelrot="0" valLabPos="-16,8" valLabRot="0" Out="true" Running="true" Voltage="5 V" Freq="22345 Hz" Always_On="true" />
<item itemtype="Oscope" CircId="Oscope-613" mainComp="false" Show_id="false" Show_Val="false" Pos="-1804,-824" rotation="0" hflip="1" vflip="1" label="Oscope-613" idLabPos="-90,-100" labelrot="0" valLabPos="-16,20" valLabRot="0" Basic_X="135 _px" Basic_Y="135 _px" BufferSize="600000 Samples" connectGnd="true" InputImped="10 MΩ" TimDiv="1000000000" TimPos="0,0,0,0," VolDiv="1,1,1,1," Tunnels=",,,," Trigger="4" Filter="0.1 V" Trigger="4" AutoSC="4" Tracks="1" HideCh="false,false,false,false," VolPos="0,0,0,0," />
<item itemtype="FreqMeter" CircId="FreqMeter-614" mainComp="false" Show_id="false" Show_Val="false" Pos="-1280,-800" rotation="0" hflip="1" vflip="1" label="FreqMeter-614" idLabPos="-32,-24" labelrot="0" valLabPos="-16,20" valLabRot="0" Filter="0.1 V" />
<item itemtype="Oscope" CircId="Oscope-624" mainComp="false" Show_id="false" Show_Val="false" Pos="-1072,-748" rotation="0" hflip="1" vflip="1" label="Oscope-624" idLabPos="-90,-100" labelrot="0" valLabPos="-16,20" valLabRot="0" Basic_X="135 _px" Basic_Y="135 _px" BufferSize="600000 Samples" connectGnd="true" InputImped="10 MΩ" TimDiv="20000000" TimPos="0,0,0,0," VolDiv="2,2,2,2," Tunnels=",,,," Trigger="0" Filter="1 V" Trigger="0" AutoSC="4" Tracks="1" HideCh="false,false,false,false," VolPos="0,0,0,0," />
<item itemtype="Node" CircId="Node-516" mainComp="false" Pos="-1076,-916" />
<item itemtype="Node" CircId="Node-519" mainComp="false" Pos="-1076,-908" />
<item itemtype="Node" CircId="Node-522" mainComp="false" Pos="-1076,-900" />
<item itemtype="Node" CircId="Node-525" mainComp="false" Pos="-1076,-892" />
<item itemtype="Node" CircId="Node-528" mainComp="false" Pos="-1076,-884" />
<item itemtype="Node" CircId="Node-531" mainComp="false" Pos="-1076,-876" />
<item itemtype="Node" CircId="Node-534" mainComp="false" Pos="-1076,-868" />
<item itemtype="Node" CircId="Node-616" mainComp="false" Pos="-1336,-896" />
<item itemtype="Node" CircId="Node-626" mainComp="false" Pos="-1336,-832" />
<item itemtype="Connector" uid="connector-535" startpinid="Resistor-34-rPin" endpinid="Led-35-rPin" pointList="-1428,-1008,-1412,-1008" />
<item itemtype="Connector" uid="connector-536" startpinid="Ground-42-Gnd" endpinid="Resistor-34-lPin" pointList="-1488,-980,-1488,-1008,-1460,-1008" />
<item itemtype="Connector" uid="connector-537" startpinid="8051-47-PORT10" endpinid="Buffer-54-in0" pointList="-1320,-1008,-1332,-1008" />
<item itemtype="Connector" uid="connector-538" startpinid="Buffer-54-out" endpinid="Led-35-lPin" pointList="-1364,-1008,-1380,-1008" />
<item itemtype="Connector" uid="connector-539" startpinid="Hd44780-57-PinEn" endpinid="Tunnel-83-pin" pointList="-1148,-1032,-1148,-992,-1116,-992" />
<item itemtype="Connector" uid="connector-540" startpinid="Tunnel-86-pin" endpinid="Hd44780-57-PinRW" pointList="-1156,-1024,-1156,-1032" />
<item itemtype="Connector" uid="connector-541" startpinid="Tunnel-85-pin" endpinid="Hd44780-57-PinRS" pointList="-1164,-1024,-1164,-1032" />
<item itemtype="Connector" uid="connector-542" startpinid="Tunnel-214-pin" endpinid="8051-47-PORT00" pointList="-1240,-1000,-1256,-1000" />
<item itemtype="Connector" uid="connector-543" startpinid="8051-47-PORT01" endpinid="Tunnel-215-pin" pointList="-1256,-992,-1240,-992" />
<item itemtype="Connector" uid="connector-544" startpinid="Tunnel-213-pin" endpinid="8051-47-PORT02" pointList="-1240,-984,-1256,-984" />
<item itemtype="Connector" uid="connector-545" startpinid="8051-47-PORT03" endpinid="Tunnel-212-pin" pointList="-1256,-976,-1240,-976" />
<item itemtype="Connector" uid="connector-546" startpinid="Tunnel-216-pin" endpinid="8051-47-PORT04" pointList="-1240,-968,-1256,-968" />
<item itemtype="Connector" uid="connector-547" startpinid="8051-47-PORT05" endpinid="Tunnel-217-pin" pointList="-1256,-960,-1240,-960" />
<item itemtype="Connector" uid="connector-548" startpinid="Tunnel-210-pin" endpinid="8051-47-PORT06" pointList="-1240,-952,-1256,-952" />
<item itemtype="Connector" uid="connector-549" startpinid="8051-47-PORT07" endpinid="Tunnel-211-pin" pointList="-1256,-944,-1240,-944" />
<item itemtype="Connector" uid="connector-550" startpinid="8051-47-PORT24" endpinid="Tunnel-247-pin" pointList="-1256,-888,-1244,-888" />
<item itemtype="Connector" uid="connector-551" startpinid="Tunnel-246-pin" endpinid="8051-47-PORT23" pointList="-1244,-880,-1256,-880" />
<item itemtype="Connector" uid="connector-552" startpinid="8051-47-PORT22" endpinid="Tunnel-245-pin" pointList="-1256,-872,-1244,-872" />
<item itemtype="Connector" uid="connector-553" startpinid="Tunnel-68-pin" endpinid="Buffer-358-in0" pointList="-972,-968,-988,-968" />
<item itemtype="Connector" uid="connector-554" startpinid="Tunnel-72-pin" endpinid="Buffer-354-in0" pointList="-972,-1000,-988,-1000" />
<item itemtype="Connector" uid="connector-555" startpinid="Buffer-355-in0" endpinid="Tunnel-67-pin" pointList="-988,-992,-972,-992" />
<item itemtype="Connector" uid="connector-556" startpinid="Tunnel-71-pin" endpinid="Buffer-356-in0" pointList="-972,-984,-988,-984" />
<item itemtype="Connector" uid="connector-557" startpinid="Buffer-357-in0" endpinid="Tunnel-70-pin" pointList="-988,-976,-972,-976" />
<item itemtype="Connector" uid="connector-558" startpinid="Tunnel-74-pin" endpinid="Buffer-353-in0" pointList="-972,-1008,-988,-1008" />
<item itemtype="Connector" uid="connector-559" startpinid="Buffer-352-in0" endpinid="Tunnel-73-pin" pointList="-988,-1016,-972,-1016" />
<item itemtype="Connector" uid="connector-560" startpinid="Tunnel-69-pin" endpinid="Buffer-351-in0" pointList="-972,-1024,-988,-1024" />
<item itemtype="Connector" uid="connector-561" startpinid="Buffer-351-out" endpinid="Hd44780-57-dataPin7" pointList="-1020,-1024,-1084,-1024,-1084,-1032" />
<item itemtype="Connector" uid="connector-562" startpinid="Buffer-352-out" endpinid="Hd44780-57-dataPin6" pointList="-1020,-1016,-1092,-1016,-1092,-1032" />
<item itemtype="Connector" uid="connector-563" startpinid="Buffer-353-out" endpinid="Hd44780-57-dataPin5" pointList="-1020,-1008,-1100,-1008,-1100,-1032" />
<item itemtype="Connector" uid="connector-564" startpinid="Buffer-354-out" endpinid="Hd44780-57-dataPin4" pointList="-1020,-1000,-1108,-1000,-1108,-1032" />
<item itemtype="Connector" uid="connector-565" startpinid="Buffer-355-out" endpinid="Hd44780-57-dataPin3" pointList="-1020,-992,-1116,-992,-1116,-1032" />
<item itemtype="Connector" uid="connector-566" startpinid="Buffer-356-out" endpinid="Hd44780-57-dataPin2" pointList="-1020,-984,-1124,-984,-1124,-1032" />
<item itemtype="Connector" uid="connector-567" startpinid="Buffer-357-out" endpinid="Hd44780-57-dataPin1" pointList="-1020,-976,-1132,-976,-1132,-1032" />
<item itemtype="Connector" uid="connector-568" startpinid="Buffer-358-out" endpinid="Hd44780-57-dataPin0" pointList="-1020,-968,-1140,-968,-1140,-1032" />
<item itemtype="Connector" uid="connector-569" startpinid="Tunnel-437-pin" endpinid="Resistor-484-lPin" pointList="-1132,-916,-1120,-916" />
<item itemtype="Connector" uid="connector-570" startpinid="Tunnel-434-pin" endpinid="Resistor-486-lPin" pointList="-1132,-908,-1120,-908" />
<item itemtype="Connector" uid="connector-571" startpinid="Tunnel-433-pin" endpinid="Resistor-487-lPin" pointList="-1132,-900,-1120,-900" />
<item itemtype="Connector" uid="connector-572" startpinid="Tunnel-432-pin" endpinid="Resistor-488-lPin" pointList="-1132,-892,-1120,-892" />
<item itemtype="Connector" uid="connector-573" startpinid="Tunnel-436-pin" endpinid="Resistor-491-lPin" pointList="-1132,-884,-1120,-884" />
<item itemtype="Connector" uid="connector-574" startpinid="Tunnel-431-pin" endpinid="Resistor-492-lPin" pointList="-1132,-876,-1120,-876" />
<item itemtype="Connector" uid="connector-575" startpinid="Tunnel-435-pin" endpinid="Resistor-489-lPin" pointList="-1132,-868,-1120,-868" />
<item itemtype="Connector" uid="connector-576" startpinid="Tunnel-438-pin" endpinid="Resistor-490-lPin" pointList="-1132,-860,-1120,-860" />
<item itemtype="Connector" uid="connector-577" startpinid="Rail-504-outnod" endpinid="Tunnel-500-pin" pointList="-1356,-1052,-1348,-1052" />
<item itemtype="Connector" uid="connector-578" startpinid="8051-47-RST" endpinid="Ground-511-Gnd" pointList="-1320,-944,-1416,-944,-1416,-936" />
<item itemtype="Connector" uid="connector-579" startpinid="Resistor-484-rPin" endpinid="Node-516-0" pointList="-1088,-916,-1076,-916" />
<item itemtype="Connector" uid="connector-580" startpinid="Resistor-486-rPin" endpinid="Node-519-0" pointList="-1088,-908,-1076,-908" />
<item itemtype="Connector" uid="connector-581" startpinid="Node-516-2" endpinid="Tunnel-513-pin" pointList="-1076,-916,-1052,-916" />
<item itemtype="Connector" uid="connector-582" startpinid="Resistor-487-rPin" endpinid="Node-522-0" pointList="-1088,-900,-1076,-900" />
<item itemtype="Connector" uid="connector-583" startpinid="Node-519-2" endpinid="Node-516-1" pointList="-1076,-908,-1076,-916" />
<item itemtype="Connector" uid="connector-584" startpinid="Resistor-488-rPin" endpinid="Node-525-0" pointList="-1088,-892,-1076,-892" />
<item itemtype="Connector" uid="connector-585" startpinid="Node-522-2" endpinid="Node-519-1" pointList="-1076,-900,-1076,-908" />
<item itemtype="Connector" uid="connector-586" startpinid="Resistor-491-rPin" endpinid="Node-528-0" pointList="-1088,-884,-1076,-884" />
<item itemtype="Connector" uid="connector-587" startpinid="Node-525-2" endpinid="Node-522-1" pointList="-1076,-892,-1076,-900" />
<item itemtype="Connector" uid="connector-588" startpinid="Resistor-492-rPin" endpinid="Node-531-0" pointList="-1088,-876,-1076,-876" />
<item itemtype="Connector" uid="connector-589" startpinid="Node-528-2" endpinid="Node-525-1" pointList="-1076,-884,-1076,-892" />
<item itemtype="Connector" uid="connector-590" startpinid="Resistor-489-rPin" endpinid="Node-534-0" pointList="-1088,-868,-1076,-868" />
<item itemtype="Connector" uid="connector-591" startpinid="Node-531-2" endpinid="Node-528-1" pointList="-1076,-876,-1076,-884" />
<item itemtype="Connector" uid="connector-592" startpinid="Resistor-490-rPin" endpinid="Node-534-1" pointList="-1088,-860,-1076,-860,-1076,-868" />
<item itemtype="Connector" uid="connector-593" startpinid="Node-534-2" endpinid="Node-531-1" pointList="-1076,-868,-1076,-876" />
<item itemtype="Connector" uid="Connector-597" startpinid="Tunnel-596-pin" endpinid="8051-47-PORT30" pointList="-1328,-936,-1320,-936" />
<item itemtype="Connector" uid="Connector-598" startpinid="8051-47-PORT31" endpinid="Tunnel-595-pin" pointList="-1320,-928,-1328,-928" />
<item itemtype="Connector" uid="Connector-612" startpinid="Clock-611-outnod" endpinid="Node-616-0" pointList="-1344,-896,-1336,-896" />
<item itemtype="Connector" uid="Connector-617" startpinid="Node-616-2" endpinid="8051-47-PORT35" pointList="-1336,-896,-1320,-896" />
<item itemtype="Connector" uid="Connector-625" startpinid="Oscope-624-Pin0" endpinid="Node-626-1" pointList="-1160,-796,-1208,-796,-1208,-768,-1336,-768,-1336,-832" />
<item itemtype="Connector" uid="Connector-627" startpinid="Node-626-2" endpinid="Node-616-1" pointList="-1336,-832,-1336,-896" />
<item itemtype="Connector" uid="Connector-615" startpinid="FreqMeter-614-lPin" endpinid="Node-626-0" pointList="-1320,-800,-1320,-832,-1336,-832" />
</circuit>

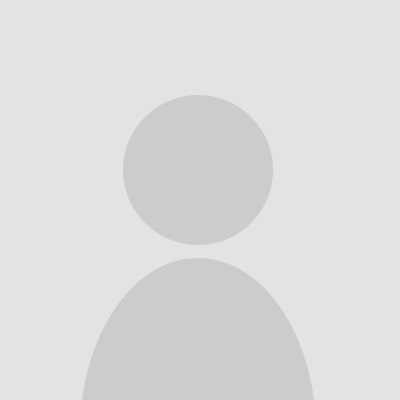
Quote from ivo on October 13, 2024, 4:04 amThankyou. That didn't seem to be an issue before. The STC89 datasheet says it has an internal pullup for EA pin.
Now I am having trouble, I can't get any print or delay to run. Often when I am stepping though debug, in my delay_ms function, SimulIDE will just crash. Is there somewhere it will show a crash log?
Thankyou. That didn't seem to be an issue before. The STC89 datasheet says it has an internal pullup for EA pin.
Now I am having trouble, I can't get any print or delay to run. Often when I am stepping though debug, in my delay_ms function, SimulIDE will just crash. Is there somewhere it will show a crash log?
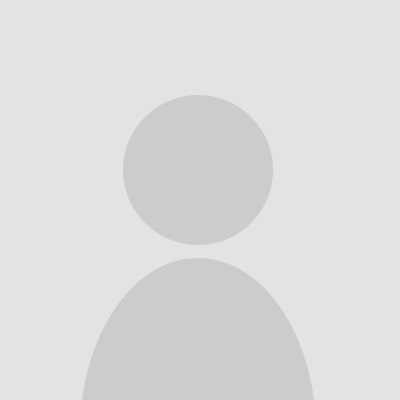
Quote from ivo on October 13, 2024, 4:16 amI modified the code to
[spoiler title=""]
int main(void) { delay_ms(1); EA = 1; // enable interrupts EX0 = 0; EX1 = 0; // Disable external interrupts IP = 0x02; // timer0 interrupt prio high IPH = 0x02; // timer0 interrupt prio highest //lcd_init(); while (1) { //lcd_clear(); //lcd_string("Counting.."); delay_ms(10); P1_0 = 0; delay_ms(10); P1_0 = 1; /*count_t1_freq(); while (counting) {} lcd_clear(); lcd_string("c: "); delay_ms(100); lcd_long(count_result); //printf_fast("%d Hz", 5); delay_ms(1000);*/ } } void delay_ms(unsigned int count) { unsigned int i, j; for (i = 0; i < count; i++) { for (j = 0; j < 2; j++) { Nop();Nop();Nop();Nop(); } Nop();Nop();Nop();Nop();Nop();Nop();Nop(); } }
[/spoiler]
If I put a breakpoint at EA = 1; then I can never step until I reach that breakpoint...
In fact it seems as if it calls the same function repeatedly? It steps into the same delay_ms function repeatedly, it never goes onto the next line of code. Like there's a phantom while loop.
I modified the code to
int main(void) {
delay_ms(1);
EA = 1; // enable interrupts
EX0 = 0; EX1 = 0; // Disable external interrupts
IP = 0x02; // timer0 interrupt prio high
IPH = 0x02; // timer0 interrupt prio highest
//lcd_init();
while (1) {
//lcd_clear();
//lcd_string("Counting..");
delay_ms(10);
P1_0 = 0;
delay_ms(10);
P1_0 = 1;
/*count_t1_freq();
while (counting) {}
lcd_clear();
lcd_string("c: ");
delay_ms(100);
lcd_long(count_result);
//printf_fast("%d Hz", 5);
delay_ms(1000);*/
}
}
void delay_ms(unsigned int count) {
unsigned int i, j;
for (i = 0; i < count; i++) {
for (j = 0; j < 2; j++) {
Nop();Nop();Nop();Nop();
}
Nop();Nop();Nop();Nop();Nop();Nop();Nop();
}
}
If I put a breakpoint at EA = 1; then I can never step until I reach that breakpoint...
In fact it seems as if it calls the same function repeatedly? It steps into the same delay_ms function repeatedly, it never goes onto the next line of code. Like there's a phantom while loop.

Quote from arcachofo on October 13, 2024, 5:43 amI didn't realize befor but you are not compiling for 8051.
There is no IPH register in 8051.If I ignore IPH and that Nop() function and compile for 8051, I get a 22.35 KHz at P3.5
I didn't realize befor but you are not compiling for 8051.
There is no IPH register in 8051.
If I ignore IPH and that Nop() function and compile for 8051, I get a 22.35 KHz at P3.5
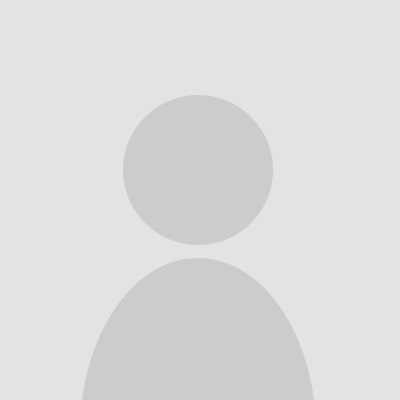
Quote from ivo on October 13, 2024, 10:06 amQuote from arcachofo on October 13, 2024, 5:43 amI didn't realize befor but you are not compiling for 8051.
There is no IPH register in 8051.If I ignore IPH and that Nop() function and compile for 8051, I get a 22.35 KHz at P3.5
True, my IRL CPU is STC89. I commented that out. Default SDCC target is a MCS51 processsor, right? But 8051 should still have NOP as valid assembly, right?
https://www.refreshnotes.com/2016/03/8051-nop-instruction.html
Quote from arcachofo on October 13, 2024, 5:43 amI didn't realize befor but you are not compiling for 8051.
There is no IPH register in 8051.If I ignore IPH and that Nop() function and compile for 8051, I get a 22.35 KHz at P3.5
True, my IRL CPU is STC89. I commented that out. Default SDCC target is a MCS51 processsor, right? But 8051 should still have NOP as valid assembly, right?
https://www.refreshnotes.com/2016/03/8051-nop-instruction.html
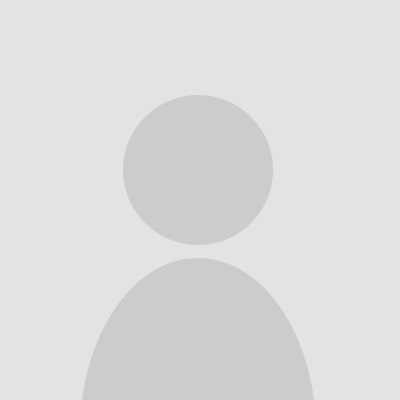
Quote from ivo on October 13, 2024, 10:14 amIn SimulIDE 1.0, I can have this code
[spoiler]
int main(void) { EA = 1; // enable interrupts EX0 = 0; EX1 = 0; // Disable external interrupts IP = 0x02; // timer0 interrupt prio high //IPH = 0x02; // timer0 interrupt prio highest, STC89 only //lcd_init(); while (1) { //lcd_clear(); //lcd_string("Counting.."); delay_ms(500); P1_0 = 0; delay_ms(500); P1_0 = 1; /*count_t1_freq(); while (counting) {} lcd_clear(); lcd_string("c: "); delay_ms(100); lcd_long(count_result); //printf_fast("%d Hz", 5); delay_ms(1000);*/ } } void delay_ms(unsigned int count) { unsigned int i, j; for (i = 0; i < count; i++) { for (j = 0; j < 35; j++) { __asm nop __endasm; __asm nop __endasm; __asm nop __endasm; __asm nop __endasm; } } }
[/spoiler]
And it works, the LED on P1_0 blinks. In SimulIDE 1.1.0, it does not blink.
In SimulIDE 1.0, I can have this code
int main(void) {
EA = 1; // enable interrupts
EX0 = 0; EX1 = 0; // Disable external interrupts
IP = 0x02; // timer0 interrupt prio high
//IPH = 0x02; // timer0 interrupt prio highest, STC89 only
//lcd_init();
while (1) {
//lcd_clear();
//lcd_string("Counting..");
delay_ms(500);
P1_0 = 0;
delay_ms(500);
P1_0 = 1;
/*count_t1_freq();
while (counting) {}
lcd_clear();
lcd_string("c: ");
delay_ms(100);
lcd_long(count_result);
//printf_fast("%d Hz", 5);
delay_ms(1000);*/
}
}
void delay_ms(unsigned int count) {
unsigned int i, j;
for (i = 0; i < count; i++) {
for (j = 0; j < 35; j++) {
__asm nop __endasm;
__asm nop __endasm;
__asm nop __endasm;
__asm nop __endasm;
}
}
}
And it works, the LED on P1_0 blinks. In SimulIDE 1.1.0, it does not blink.

Quote from arcachofo on October 13, 2024, 10:23 amIt is working for me:
#include <8051.h> void delay_ms(unsigned int count) { unsigned int i, j; for (i = 0; i < count; i++) { for (j = 0; j < 35; j++) { __asm nop __endasm; __asm nop __endasm; __asm nop __endasm; __asm nop __endasm; } } } int main(void) { EA = 1; // enable interrupts EX0 = 0; EX1 = 0; // Disable external interrupts IP = 0x02; // timer0 interrupt prio high //IPH = 0x02; // timer0 interrupt prio highest, STC89 only //lcd_init(); while (1) { //lcd_clear(); //lcd_string("Counting.."); delay_ms(500); P1_0 = 0; delay_ms(500); P1_0 = 1; /*count_t1_freq(); while (counting) {} lcd_clear(); lcd_string("c: "); delay_ms(100); lcd_long(count_result); //printf_fast("%d Hz", 5); delay_ms(1000);*/ } }
It is working for me:
#include <8051.h>
void delay_ms(unsigned int count) {
unsigned int i, j;
for (i = 0; i < count; i++) {
for (j = 0; j < 35; j++) {
__asm nop __endasm;
__asm nop __endasm;
__asm nop __endasm;
__asm nop __endasm;
}
}
}
int main(void) {
EA = 1; // enable interrupts
EX0 = 0; EX1 = 0; // Disable external interrupts
IP = 0x02; // timer0 interrupt prio high
//IPH = 0x02; // timer0 interrupt prio highest, STC89 only
//lcd_init();
while (1) {
//lcd_clear();
//lcd_string("Counting..");
delay_ms(500);
P1_0 = 0;
delay_ms(500);
P1_0 = 1;
/*count_t1_freq();
while (counting) {}
lcd_clear();
lcd_string("c: ");
delay_ms(100);
lcd_long(count_result);
//printf_fast("%d Hz", 5);
delay_ms(1000);*/
}
}

Quote from arcachofo on October 13, 2024, 10:27 amThimking about it, maybe you should try the last build:
https://simulide.com/p/testers/
Thimking about it, maybe you should try the last build:
https://simulide.com/p/testers/