I2C To Parallel in AVR
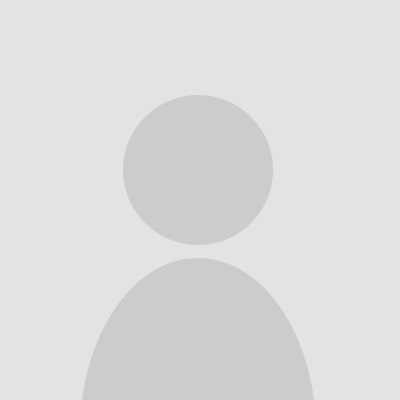
Quote from lvivgeorge on November 5, 2024, 8:20 pmPlease help me understand what I'm doing wrong here.
I've tested with a real Arduino UNO (compiled using raw AVR) and a PCF8574 (as module for LCD1602, and simply connected to P0, P1 and P2), and everything works perfectly.
I want to reproduce same example in Simulide, but it's not working, I made next circuit, changed I2C address to 63 in I2C expander, but nothing happens
My code
#include <avr/io.h>
#include <util/delay.h>
#include <avr/interrupt.h>
#define F_CPU 16000000UL // CPU frequency
#define PCF8574_ADDRESS 0x3F
void i2cInit();
void i2cStart();
void i2cStop();
void i2cWrite(uint8_t data);
void PCF8574_write(uint8_t data) {
i2cStart();
i2cWrite(PCF8574_ADDRESS<<1); // Left-shift to add write bit
i2cWrite(data); // Send data to PCF8574
i2cStop();
}
int main(void) {
i2cInit();
while (1) {
PCF8574_write(0x00);
_delay_ms(500);
PCF8574_write(0xFF);
_delay_ms(500);
}
return0;
}
void i2cInit() {
TWSR=0x00; // Set prescaler to 1
TWBR=0x48; // 100kHz SCL frequency for F_CPU = 16MHz
TWCR= (1<<TWEN); // Enable TWI
}
void i2cStart() {
TWCR= (1<<TWINT) | (1<<TWSTA) | (1<<TWEN); // Send start condition
while (!(TWCR& (1<<TWINT))); // Wait for TWINT flag
_delay_us(10);
}
void i2cStop() {
TWCR= (1<<TWINT) | (1<<TWSTO) | (1<<TWEN); // Send stop condition
_delay_us(10);
}
void i2cWrite(uint8_t data) {
TWDR=data;
TWCR= (1<<TWINT) | (1<<TWEN); // Start transmission
while (!(TWCR& (1<<TWINT))); // Wait for TWINT flag
}
Please help me understand what I'm doing wrong here.
I've tested with a real Arduino UNO (compiled using raw AVR) and a PCF8574 (as module for LCD1602, and simply connected to P0, P1 and P2), and everything works perfectly.
I want to reproduce same example in Simulide, but it's not working, I made next circuit, changed I2C address to 63 in I2C expander, but nothing happens
My code
#include <avr/io.h>
#include <util/delay.h>
#include <avr/interrupt.h>
#define F_CPU 16000000UL // CPU frequency
#define PCF8574_ADDRESS 0x3F
void i2cInit();
void i2cStart();
void i2cStop();
void i2cWrite(uint8_t data);
void PCF8574_write(uint8_t data) {
i2cStart();
i2cWrite(PCF8574_ADDRESS<<1); // Left-shift to add write bit
i2cWrite(data); // Send data to PCF8574
i2cStop();
}
int main(void) {
i2cInit();
while (1) {
PCF8574_write(0x00);
_delay_ms(500);
PCF8574_write(0xFF);
_delay_ms(500);
}
return0;
}
void i2cInit() {
TWSR=0x00; // Set prescaler to 1
TWBR=0x48; // 100kHz SCL frequency for F_CPU = 16MHz
TWCR= (1<<TWEN); // Enable TWI
}
void i2cStart() {
TWCR= (1<<TWINT) | (1<<TWSTA) | (1<<TWEN); // Send start condition
while (!(TWCR& (1<<TWINT))); // Wait for TWINT flag
_delay_us(10);
}
void i2cStop() {
TWCR= (1<<TWINT) | (1<<TWSTO) | (1<<TWEN); // Send stop condition
_delay_us(10);
}
void i2cWrite(uint8_t data) {
TWDR=data;
TWCR= (1<<TWINT) | (1<<TWEN); // Start transmission
while (!(TWCR& (1<<TWINT))); // Wait for TWINT flag
}

Quote from arcachofo on November 5, 2024, 9:19 pmHi.
There are 2 things that can help:
1- Add pullup resistors to I2C lines.
2- Drive the LEDs as Common Anode.
I2C to Parallel has open collector outputs with weak pullups, so to drive LEDs you need some kind of driver or use as Common Anode (you will see LEDs On at Low and Off at High).
Hi.
There are 2 things that can help:
1- Add pullup resistors to I2C lines.
2- Drive the LEDs as Common Anode.
I2C to Parallel has open collector outputs with weak pullups, so to drive LEDs you need some kind of driver or use as Common Anode (you will see LEDs On at Low and Off at High).
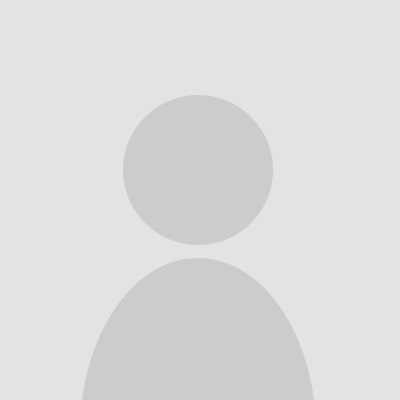
Quote from lvivgeorge on November 6, 2024, 6:36 amThanks a lot! now it works!
Thanks a lot! now it works!